伟大的阿尔伯特-爱因斯坦说过–‘永远不要记住那些你可以查到的东西’。
有了这些唾手可得的 Python 代码片段,还需要记住每一种编码解决方案吗?
1.将多个数据框合并为一个
我们经常会收到多个文件中的数据,为了有效地进行分析,必须将这些数据合并到一个数据框中。
import pandas as pd# Sample DataFramesdf1 = pd.DataFrame({'ID': [1, 2, 3], 'Name': ['Alice', 'Bob', 'Charlie']})df2 = pd.DataFrame({'ID': [2, 3, 4], 'Age': [25, 30, 35]})df3 = pd.DataFrame({'ID': [1, 2], 'City': ['New York', 'Los Angeles']})# 方法 1: 将 df1 和 df2 根据 ID 列进行合并。on='ID':指定根据哪个列(ID 列)进行合并。how='inner':使用 INNER JOIN,即只保留两个 DataFrame 中 ID 列相匹配的行。merged_df = pd.merge(df1, df2, on='ID', how='inner')# 方法 2: 先将 df1 和 df2 的 ID 列设置为索引,然后使用 join 方法基于索引进行连接。joined_df = df1.set_index('ID').join(df2.set_index('ID'))# 方法 3: 将 df1 和 df3 沿着行的方向拼接(相当于竖向叠加),即将两者的行组合起来。concatenated_df = pd.concat([df1, df3], ignore_index=True)print('Merged DataFrame:n', merged_df)print('nJoined DataFrame:n', joined_df)print('nConcatenated DataFrame:n', concatenated_df)
2.列表扁平化
列表扁平化涉及将嵌套列表转换为单个统一的列表。列表推导式使这一过程既简单又高效,从而简化了数据的操作和访问。
nested_list = [[1, 2, 3], [4, 5], [6, 7], [8,9],[10,11]]flattened_list = [item for sublist in nested_list for item in sublist]print(flattened_list)'''Output'''[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
3.在 Pandas 中根据日期范围筛选数据
处理时间序列数据通常需要过滤特定日期范围内的记录。例如,要检索 2021 年 1 月 1 日至 2022 年 1 月 1 日之间的数据,可以使用带有布尔索引的 `.loc` 访问器。这种方法提供了一种简洁高效的按日期过滤数据的方法。
import pandas as pd# Sample DataFrame with a Date columndata = { 'Date': ['2021-01-15', '2021-02-05', '2021-05-20', '2021-12-25', '2022-02-01', '2022-03-10'], 'Value': [10, 20, 30, 40, 50, 60]}df = pd.DataFrame(data)# Convert 'Date' column to datetime formatdf['Date'] = pd.to_datetime(df['Date'])# Define the date rangestart_date = '2021-01-01'end_date = '2022-01-01'# Filter the DataFrame for the specified date rangefiltered_df = df.loc[(df['Date'] >= start_date) & (df['Date'] <= end_date)]print(filtered_df)
4.将月数据转换为日或周数据
在处理按月报告的数据时,你可能需要将其转换为以日或周为间隔的数据,以便更好地进行分析。
import pandas as pd# Sample DataFrame with monthly datadates = pd.date_range('2021-01-01', periods=4, freq='M')data = pd.DataFrame({'Value': [100, 200, 300, 400]}, index=dates)# Method 1: Convert Monthly to Dailydaily_data = data.resample('D').ffill()# Method 2: Convert Monthly to Weeklyweekly_data = data.resample('W').ffill()print('Daily Data:n', daily_data)print('nWeekly Data:n', weekly_data)
5.切片
在 Python 中,切片是一项常见任务。通过切片,你可以轻松提取列表或字符串的特定部分。
# Sample listdata = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]# 1. Reversing a listreversed_data = data[::-1]print('Reversed:', reversed_data)# 2. Slicing with a stepstep_slice = data[::2] # Every second elementprint('Every second element:', step_slice)# 3. Slicing with a negative step (reverse slice)reverse_step_slice = data[8:2:-2] # Slices from index 8 to 2 with step -2print('Reverse step slice:', reverse_step_slice)# 4. Slicing with start and end indicesrange_slice = data[3:7] # Elements from index 3 to 6print('Elements from index 3 to 6:', range_slice)# 5. Slicing with a combination of start, end, and stepcombined_slice = data[1:9:3] # Elements from index 1 to 8 with step 3print('Elements from index 1 to 8 with step 3:', combined_slice)
6.字典列表排序
当你有一个字典列表时,按特定键排序可以帮助你更有效地组织数据。如下述代码可方便地根据日期、姓名或数值等标准排列数据。
dict1 = [ {'Name':'Karl', 'Age':25}, {'Name':'Lary', 'Age':39}, {'Name':'Nina', 'Age':35}]## Using sort()dict1.sort(key=lambda item: item.get('Age'))# List sorting using itemgetterfrom operator import itemgetterf = itemgetter('Name')dict1.sort(key=f)# Iterable sorted functiondict1 = sorted(dict1, key=lambda item: item.get('Age'))'''Output[{'Age': 25, 'Name': 'Karl'}, {'Age': 35, 'Name': 'Nina'}, {'Age': 39, 'Name': 'Lary'}]'''
7.获取每列中缺失值的计数
缺失数据是数据科学项目中的一个主要问题,处理它是最重要的任务之一。下述代码段可快速统计每一列中的缺失值,从而更容易识别不完整数据并决定如何处理。
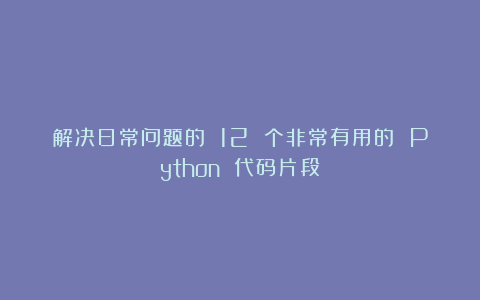
import pandas as pddf = pd.read_csv('https://raw./Abhayparashar31/datasets/refs/heads/master/titanic.csv')missing_values_count = df.isnull().sum()print('Count of Missing Values in Each Column:')print(missing_values_count)
8.将分类数据转换为整数
将分类数据转换为整数是为机器学习模型做准备的常见步骤。下述代码段简化了这一过程,使数据更容易用于需要数字输入的算法中。
import pandas as pd# Sample DataFrame with categorical datadf = pd.DataFrame({ 'Category': ['A', 'B', 'A', 'C', 'B', 'C']})# Method 1: Using pd.factorize()df['Category_Factorize'] = pd.factorize(df['Category'])[0]# Method 2: Using pd.Categoricaldf['Category_Categorical'] = pd.Categorical(df['Category']).codes# Method 3: Using LabelEncoder from sklearnfrom sklearn.preprocessing import LabelEncoderle = LabelEncoder()df['Category_LabelEncoder'] = le.fit_transform(df['Category'])print(df)
9.精确的浮点数格式化
精确的浮点数格式化对于准确、清晰地显示数值数据至关重要。
# 1. Using f-strings (formatted string literals)value = 123.456789formatted_value = f'{value:.2f}' # Keeps 2 decimal placesprint(formatted_value) # Output: 123.46# 2. Using format() methodformatted_value = '{:.2f}'.format(value) # Format with 2 decimal placesprint(formatted_value) # Output: 123.46# 3. Using round() functionrounded_value = round(value, 2)print(rounded_value) # Output: 123.46# 4. Using % string formattingformatted_value = '%.2f' % value # Format to 2 decimal placesprint(formatted_value) # Output: 123.46# 5. Using Decimal for precise decimal handlingfrom decimal import Decimalvalue = Decimal('123.456789')formatted_value = round(value, 2)print(formatted_value) # Output: 123.46
10.处理英式日期格式列
许多国家使用英式日期格式,即日期以日-月-年的形式书写。你可以启用 dayfirst 参数来处理这种格式。
import pandas as pddf = pd.DataFrame({'date_time': ['6/12/2000 12:32', '13/5/2001 11:45', '11/12/2005 1:57'], 'value': [2, 3, 4]})pd.to_datetime(df['date_time'],dayfirst=True)
11.将 JSON 转换为 CSV
处理 JSON 格式的数据很常见,但许多工具和应用程序更喜欢 CSV 文件。下述代码段解决了将 JSON 数据转换为 CSV 的日常难题,使其更易于使用电子表格或执行数据分析任务。
import pandas as pdfrom pandas import json_normalizeimport json# Load JSON datawith open('/content/data.json') as f: ##Colab Sample JSOn data = json.load(f)# Normalize the JSON datadf = json_normalize(data)# Save to CSVdf.to_csv('output_file.csv', index=False)
12.用一行查找集合的所有子集
在处理集合时,一项常见的任务就是找到所有可能的子集,无论是生成用于测试、数学计算的组合,还是解决算法问题。
下述代码段只需一行代码就能解决查找集合所有子集的日常难题。在需要的时候,它是一个方便的工具。
from itertools import combinationsdata = [55,65,32,44,67]print(list(combinations(data, 3)))'''OUTPUT'''[(55, 65, 32), (55, 65, 44), (55, 65, 67), (55, 32, 44), (55, 32, 67), (55, 44, 67), (65, 32, 44), (65, 32, 67), (65, 44, 67), (32, 44, 67)]